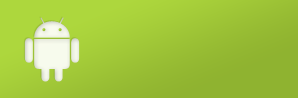
AndroidのActivityInstrumentationTestCase2でUIをテストしました
ActivityInstrumentationTestCase2というテストケースクラスを使って UIの機能をテストできるみたいなので試しました。
以下はテスト対象になるサンプルアプリです。 ボタンを押すとTextViewに"Hello World!"と表示するだけのアプリです。
◎レイアウト
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/button1" android:text="Button" android:layout_width="fill_parent" android:layout_height="wrap_content" android:onClick="onClick"/> <TextView android:id="@+id/textView1" android:layout_width="fill_parent" android:layout_height="wrap_content"/> </LinearLayout>
◎アクティビティ
package sample.button; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.TextView; public class SampleButton extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); } public void onClick(View v) { TextView textView1 = (TextView) findViewById(R.id.textView1); textView1.setText("Hello World!"); } }
次はJUnitテストケースを作成になります。 ここでスーパークラスをActivityInstrumentationTestCase2にして下さい。 場所はSampleButtonと同じところに作成しました。 ボタンを押した際にTextViewの文字列が"Hello World!"になるかテストしています。
◎テストクラス
package sample.button; import android.test.ActivityInstrumentationTestCase2; import android.widget.Button; import android.widget.TextView; public class SampleButtonTest extends ActivityInstrumentationTestCase2<SampleButton> { private TextView textView1; private Button button1; public SampleButtonTest() { super("sample.button", SampleButton.class); } @Override protected void setUp() throws Exception { super.setUp(); button1 = (Button) getActivity().findViewById(R.id.button1); textView1 = (TextView) getActivity().findViewById(R.id.textView1); } public void testOnClick() { getActivity().runOnUiThread( new Runnable() { @Override public void run() { // ボタンを押す button1.performClick(); } }); // 待機する getInstrumentation().waitForIdleSync(); // TextViewに表示された文字列を確認する assertEquals("Hello World!", textView1.getText()); } }
最後にAndroidManifest.xmlを修正します。 uses-libraryタグとinstrumentationタグを追加しています。 それ以外はデフォルトです。 ◎AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="sample.button" android:versionCode="1" android:versionName="1.0"> <application android:icon="@drawable/icon" android:label="@string/app_name"> <activity android:name="SampleButton" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <uses-library android:name="android.test.runner" /> </application> <instrumentation android:name="android.test.InstrumentationTestRunner" android:targetPackage="sample.button"/> </manifest>
準備ができたのでAndroid JUnit Testで起動して下さい。
JUnitビューを開いて以下のように緑のラインが表示されたら成功です。